Flutter开发中的页面跳转和传值
条评论在Android原生开发中,页面跳转用Intent类实现
1 | Intent intent =new Intent(MainActivity.this,SecondActivity.class); |
而在安卓原生开发中,页面传值有多种方法,常见的可以用intent、Bundle、自定义类、静态变量等等来传值。
Flutter提供了两种方法路由,分别是 Navigator.push() 以及 Navigator.pushNamed() 。
此文基于 Flutter版本 Channel stable,v1.9.1+hotfix.2
页面跳转
构建路由Navigator.push()
- Navigator.push()
从第一个页面(FirstPage())跳转到第二个页面(SecondPage())1
2
3
4
5
6
7// Within the `FirstPage` widget
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SecondPage()),
);
} - 使用
Navigator.pop()
回到第一个页面(FirstPage())1
2
3
4// Within the SecondPage widget
onPressed: () {
Navigator.pop(context);
}
命名路由Navigator.pushNamed()
- Navigator.pushNamed()
首先需要定义一个routes
1
2
3
4
5
6
7
8
9
10
11
12
13MaterialApp(
// home: FirstPage(),
// Start the app with the "/" named route. In this case, the app starts
// on the FirstPage widget.
initialRoute: '/',
routes: {
// When navigating to the "/" route, build the FirstPage widget.
'/': (context) => FirstPage(),
// When navigating to the "/second" route, build the SecondPage widget.
'/second': (context) => SecondPage(),
},
);
注意这里定义了
initialRoute
之后,就不能定义home
属性。应该把之前定义的home
属性注释掉。initialRoute
属性不能与home
共存,只能选一个。
- 从第一个页面(FirstPage())跳转到第二个页面(SecondPage())
1 | // Within the `FirstPage` widget |
使用Navigator.pop()
回到第一个页面(FirstPage())
1 | // Within the SecondPage widget |
传值跳转
构建路由Navigator.push()
- 首先定义需要传的值
1 | // You can pass any object to the arguments parameter. |
- 第二个页面(SecondPage())接受传值
1 | // A widget that extracts the necessary arguments from the ModalRoute. |
- 从第一个页面(FirstPage())传值
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19onPressed: () {
// When the user taps the button, navigate to the specific route
// and provide the arguments as part of the RouteSettings.
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => SecondPage(),
// Pass the arguments as part of the RouteSettings. The
// ExtractArgumentScreen reads the arguments from these
// settings.
settings: RouteSettings(
arguments: ScreenArguments(
'Extract Arguments Screen',
'This message is extracted in the build method.',
),
),
),
);
}
命名路由Navigator.pushNamed()
- 首先定义需要传的值
1 | // You can pass any object to the arguments parameter. |
其次定义一下
routes
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24MaterialApp(
// Provide a function to handle named routes. Use this function to
// identify the named route being pushed, and create the correct
// Screen.
onGenerateRoute:
(settings) {
// If you push the PassArguments route
if (settings.name == "/passArguments") {
// Cast the arguments to the correct type: ScreenArguments.
final ScreenArguments args = settings.arguments;
// Then, extract the required data from the arguments and
// pass the data to the correct screen.
return MaterialPageRoute(
builder: (context) {
return SecondPage(
title: args.title,
message: args.message,
);
},
);
}
},
);第二个页面(SecondPage())接受传值
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28// A Widget that accepts the necessary arguments via the constructor.
class SecondPage extends StatelessWidget {
final String title;
final String message;
// This Widget accepts the arguments as constructor parameters. It does not
// extract the arguments from the ModalRoute.
//
// The arguments are extracted by the onGenerateRoute function provided to the
// MaterialApp widget.
const SecondPage({
Key key,
this.title,
this.message,
}) : super(key: key);
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(title),
),
body: Center(
child: Text(message),
),
);
}
}从第一个页面(FirstPage())传值
1
2
3
4
5
6
7
8
9
10
11
12onPressed: () {
// When the user taps the button, navigate to a named route
// and provide the arguments as an optional parameter.
Navigator.pushNamed(
context,
"/passArguments",
arguments: ScreenArguments(
'Accept Arguments Screen',
'This message is extracted in the onGenerateRoute function.',
),
);
}
第三方插件
Fluro是Flutter路由库
添加方式
1 | dependencies: |
使用例子
1 | import 'package:flutter/material.dart'; |
‘app_route.dart’的代码:
1 | import 'package:fluro/fluro.dart'; |
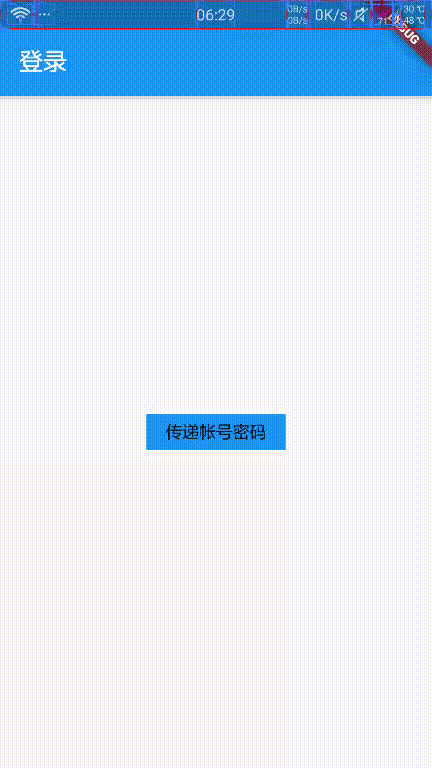
Reference
本文标题:Flutter开发中的页面跳转和传值
文章作者:xmaihh
发布时间:2019-10-13
最后更新:2019-10-13
原始链接:https://xmaihh.github.io/blog/2019/10/13/flutter-kai-fa-zhong-de-ye-mian-tiao-zhuan-he-chuan-zhi/
版权声明:采用[CC BY-NC-SA 4.0许可协议]进行许可
分享